Building Web Application with React + Apollo Client + GraphQL + .NET Core WebAPI
This article is really about showing you, how we can build a web application using React, GraphQL and .NET Core WebAPI. I would like to think of this as a Tutorial cum Sharing/Post-Mortem session after working through all those frustrated moments throughout the entire course. The demo application's source codes can be found on GitHub https://github.com/DriLLFreAK100/graphql-dotnet-poc
Background
Back when I was building the demo application (somewhere back in early August 2020), the GraphQL.NET version 3.x was still in the preview stages. Many complimentary packages were not up supporting it yet. Therefore, after many trials and errors, I have decided to work with its stable release, version 2.4.0 instead. So yes, if you are following through this tutorial, you need to have .NET Core 2.2 SDK being installed on your machine first as the demo is developed with .NET Core 2.2 as Traget framework.
Motivation
The real motivation for me to write this article, is really to help the folks out there struggling to find a COMPLETE guide or tutorial on working with GraphQL.NET. Not to mention, the resources found on the web are also very limited on this topic. It's either the tutorial/example is not complete or there isn't any source code repo provided for us to refer to.
Also, GraphQL Subscription topic is something that's almost missing in the entire web (For .NET only of course!). There are some real sneaky CAVEATS to it. So, just for the benefits of all the other .NET folks out there struggling to get this part up just like I did, don't worry, I've got you covered here mates! 😉
Topics Coverage
Please feel free to skip to the sections of your interest.
- What is GraphQL?
- What's NOT GraphQL?
- Where does GraphQL sits in a Software Architecture?
- Configuring GraphQL Server
- Building GraphQL Schema
- Write and Test GraphQL queries with GraphiQL
- React + Apollo Client Integration
- Demo - Query, Mutation and Subscription in Action!
- Caveat!
1. What is GraphQL
Based on the official graphql.org site's definition, GraphQL is
A query language for your API
GraphQL is a query language for APIs and a runtime for fulfilling those queries with your existing data. GraphQL provides a complete and understandable description of the data in your API, gives clients the power to ask for exactly what they need and nothing more, makes it easier to evolve APIs over time, and enables powerful developer tools.
As mentioned in https://graphql.org/
If I were to rephrase and lamen-ize it, it is basically a Query Language or a Payload format that a Client Application has to conform to when making a request to the GraphQL Server. In a HTTP Context, this is referring to the Payloads contained in a POST Request body.
A Query Language or a Payload format that a Client Application has to conform to when making a request to the GraphQL Server.
My lamen half-baked take on its definition
2. What's NOT GraphQL?
If you don't bother to even do a basic read up on this topic, GraphQL could possibly be a technology related to Database since there is the QL in its name. Truth is that, GraphQL has zero thing to do with Database at all. I will show you that in the next section, where I explain its placement in a Software Architecture stack.
Another common misconception is that GraphQL enables Client Apps to query the API however it likes and things will happen magically.
Truth is that, it is not a new protocol and there are still works needed to be done in order for the magic to happen. In order for Client Apps to make a query to backend successfully, GraphQL Server has to be coded, programmed, expose or make those set of resources (graph) available to the clients first!
3. Where does GraphQL sits in a Software Architecture?
Let us use the classic 3-tier architecture as an example - Presentation (Client), Domain (Server) and Data layers. Where does GraphQL actually sits in here?
Well, it actually sits between Client and Server. The diagram below pretty much sums it all up.
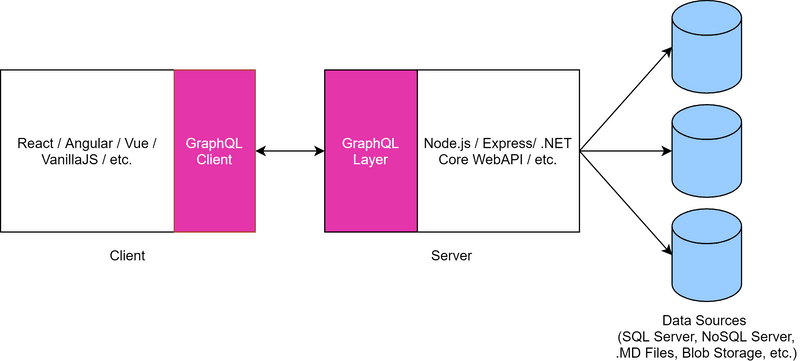
The GraphQL Layer highlighted in the Server tier, is actually a layer that provides capability to the API Server to make it a GraphQL Server. Read that again, let that sink.
Usually, we do not reinvent the wheel. Chances is, there is already package available for whichever language or stack that you are using. For Node.js, people usually use express-graphql. For .NET Core, people usually use graphql-dotnet. It contains all those heavy liftings like parsing the GraphQL queries sent from Client applications, etc. You can refer here for more information on other languages implementing GraphQL Server.
4. Configuring GraphQL Server
Alright, enough introduction from me! Let's get into the practical stuffs! We will start by setting up our GraphQL Server in a .NET Core WebAPI application.
I will only mention the gist of it here. If you are just starting out or are confused with which packages to be installed where, I will strongly suggest that you clone the repo to your local machine and open the .sln solution file in the Demo.Server folder with Visual Studio. That will give you a working example to begin with, rather than digging the solutions elsewhere.
I have setup 2 projects in my solution, a WebAPI and a Class Library project, both targeting .NET Core 2.2 as Target framework in their properties.
I'm actually taking reference to the Clean Architecture here for the code organization. And for the simplicity sake of this demo, I have excluded the Infrastructure layer as the demonstration of GraphQL's capabilities doesn't really require that.
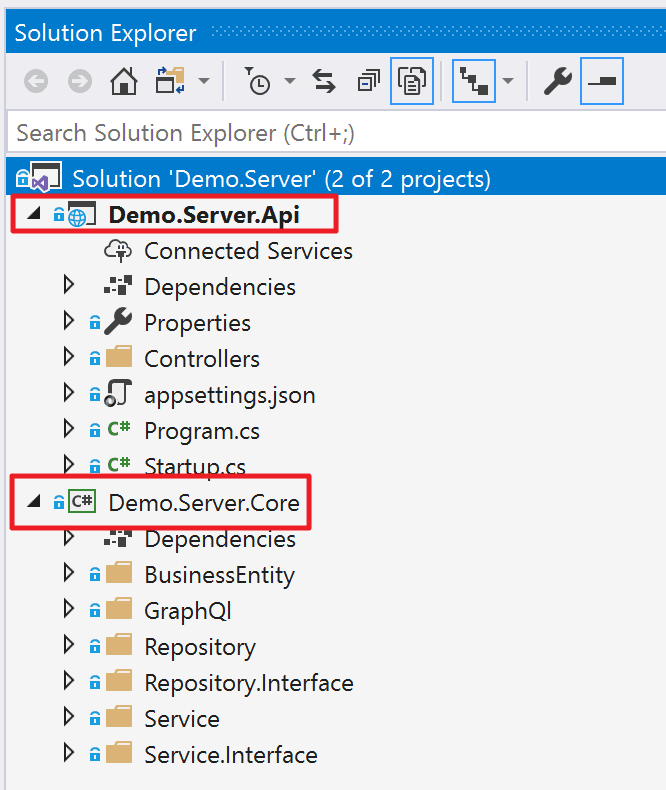
Let's start with the Startup.cs file. There are 2 things that are crucial to get things work properly here. First and foremost, it would the ConfigureServices method. We need to include the dependency injection configs like how we usually do it for a .NET Core web application. To make things more clear cut, I have grouped all the GraphQL related DI into a method called ConfigureGraphQlScopes. Do pay attention to that AddWebSockets() extension method here. That is crucial to get the GraphQL Subscription to work as it relies on WebSocket protocol.
1public void ConfigureGraphQlScopes(IServiceCollection services)
2{
3 services.AddSingleton<IDocumentExecuter, DocumentExecuter>();
4 services.AddSingleton<IDocumentWriter, DocumentWriter>();
5 services.AddSingleton<ClientType>();
6 services.AddSingleton<FeedbackInputType>();
7 services.AddSingleton<FeedbackType>();
8 services.AddSingleton<FeedbackEventType>();
9 services.AddSingleton<TransactionType>();
10 services.AddSingleton<MonthType>();
11 services.AddSingleton<TransactionCategoryType>();
12 services.AddSingleton<TransactionSummaryByMonthType>();
13 services.AddSingleton<ClientTransactionSummaryDtoType>();
14 services.AddSingleton<DemoQuery>();
15 services.AddSingleton<DemoMutation>();
16 services.AddSingleton<DemoSubscription>();
17 services.AddSingleton<ISchema, DemoSchema>();
18 services.AddSingleton<IDependencyResolver>(d => new FuncDependencyResolver(d.GetRequiredService));
19
20 services
21 .AddGraphQL(new GraphQLOptions()
22 {
23 EnableMetrics = true,
24 ExposeExceptions = true
25 })
26 .AddWebSockets()
27 .AddDataLoader()
28 .AddGraphTypes();
29}
30
Second part would be the Configure method where the HTTP middleware is configured. Over here, we need to configure the WebSockets middleware (for Subscription to work) and also the endpoint configurations for the GraphQL API and GraphiQL (Test tool for GraphQL queries).
1public void Configure(IApplicationBuilder app, IHostingEnvironment env)
2{
3 app.UseCors("AllowAllOrigins");
4 if (env.IsDevelopment())
5 {
6 app.UseDeveloperExceptionPage();
7 }
8 else
9 {
10 // The default HSTS value is 30 days. You may want to change this for production scenarios, see https://aka.ms/aspnetcore-hsts.
11 app.UseHsts();
12 }
13
14 app.UseWebSockets();
15 app.UseGraphQLWebSockets<ISchema>("/graphql");
16
17 app.UseGraphiQLServer(new GraphiQLOptions()
18 {
19 GraphiQLPath = "/graphiql",
20 GraphQLEndPoint = "/graphql"
21 });
22 app.UseHttpsRedirection();
23 app.UseMvc();
24}
25
Next would be the single HTTP POST endpoint that handles all the GraphQL requests. For that, I have setup a GraphQlController with a single POST method in it. The method basically invokes the GraphQL.NET package's DocumentExecuter function along with the request payloads.
1[Route("graphql")]
2public class GraphQlController : ControllerBase
3{
4 private readonly ISchema _schema;
5 private readonly IDocumentExecuter _executer;
6 private readonly IDocumentWriter _writer;
7
8 public GraphQlController(IDocumentExecuter executer, IDocumentWriter writer, ISchema schema)
9 {
10 _executer = executer;
11 _writer = writer;
12 _schema = schema;
13 }
14
15 public async Task<IActionResult> Post([FromBody] GraphQLQuery query)
16 {
17 var result = await _executer.ExecuteAsync(_ =>
18 {
19 _.Schema = _schema;
20 _.Query = query.Query;
21 _.OperationName = query.OperationName;
22 _.Inputs = query.Variables.ToInputs();
23 });
24
25 if (result.Errors?.Count > 0)
26 {
27 return BadRequest();
28 }
29
30 return Ok(result);
31 }
32}
33
Cool, we are now done with all the configurations and setups required for our .NET Core WebAPI GraphQL Server! Up next, we will focus on writing the Schema for our GraphQL Server.
5. Building GraphQL Schema
As mentioned on GraphQL.NET Docs, there are 2 ways to build Schema, i.e. Schema-first or Code-first (GraphType-first). Sounds familiar eh? Yep, the semantics are taken from ORMs like Entity Framework. In my demo, the schema is built via Code-first approach, as I felt more natural that way. I have no comment on which is better over the other. To me, getting things done and feeling comfortable is more important. So yea, whichever method that works best for you!
I have included all my GraphQL Schema related classes in Demo.Server.Core project > GraphQl folder. There are 2 subfolders within it, the Schema and Types folder. I would like to think of it this way, classes in the Schema folder are the blueprint classes of my GraphQL Server, the roots of my graphs - the Query, Mutation and Subscription.
The DemoSchema.cs is like my registrar for Query, Mutation and Subscription. Let's take a look at it together. It is inheriting the GraphQL.Types.Schema class, which enables us to specify the Query, Mutation, Subscription, DependencyResolver, which are all injected based on the configuration in Startup.cs
1using GraphQL;
2
3namespace Demo.Server.Core.GraphQl.Schema
4{
5 public class DemoSchema: GraphQL.Types.Schema
6 {
7 public DemoSchema(DemoQuery query, DemoMutation mutation, DemoSubscription subscription, IDependencyResolver resolver)
8 {
9 Query = query;
10 Mutation = mutation;
11 Subscription = resolver.Resolve<DemoSubscription>();
12 DependencyResolver = resolver;
13 }
14 }
15}
16
How things are hooked up? Well, there really are no magics to it. If you take a closer look at the Startup.cs, in the DI section, ISchema is configured with DemoSchema as its implementation class. And in the GraphQlController class, ISchema is injected into it and being passed into the ExecuteAsync function from DocumentWriter. That is how it identifies the Schema.
There is one thing to be kept in mind, in order to expose something in the Schema, it has to inherit GraphType. So, some rule goes for the Query, Mutation and Subscription root classes. In the example I provided, they are all inheriting the ObjectGraphType.
Query
1using Demo.Server.Core.GraphQl.Types;
2using Demo.Server.Core.Service.Interface;
3using GraphQL.Types;
4
5namespace Demo.Server.Core.GraphQl.Schema
6{
7 public class DemoQuery : ObjectGraphType<object>
8 {
9 public DemoQuery(ITransactionService transactionService, IClientService clientService)
10 {
11 Name = "Query";
12 Field<ListGraphType<TransactionType>>(
13 "transactions",
14 arguments: new QueryArguments(new QueryArgument<NonNullGraphType<IntGraphType>> { Name = "year" }),
15 resolve: context => transactionService.GetTransactions(context.GetArgument<int>("year")),
16 description: "Get all transactions for the specified year"
17 );
18 Field<ListGraphType<ClientType>>(
19 "clients",
20 resolve: _ => clientService.GetClients(),
21 description: "Get all available clients"
22 );
23 Field<ListGraphType<ClientType>>(
24 "client",
25 arguments: new QueryArguments(new QueryArgument<NonNullGraphType<IntGraphType>> { Name = "id" }),
26 resolve: context => clientService.GetClient(context.GetArgument<int>("id")),
27 description: "Get client with specified ID"
28 );
29 Field<ListGraphType<ClientTransactionSummaryDtoType>>(
30 "topPerformingClient",
31 arguments: new QueryArguments(new QueryArgument<NonNullGraphType<IntGraphType>> { Name = "count" }, new QueryArgument<NonNullGraphType<IntGraphType>> { Name = "year" }),
32 resolve: context => clientService.GetTopPerformingClients(context.GetArgument<int>("count"), context.GetArgument<int>("year")),
33 description: "Get top performing clients. Max limit count = 5"
34 );
35 Field<ListGraphType<ClientTransactionSummaryDtoType>>(
36 "worstPerformingClient",
37 arguments: new QueryArguments(new QueryArgument<NonNullGraphType<IntGraphType>> { Name = "count" }, new QueryArgument<NonNullGraphType<IntGraphType>> { Name = "year" }),
38 resolve: context => clientService.GetWorstPerformingClients(context.GetArgument<int>("count"), context.GetArgument<int>("year")),
39 description: "Get worst performing clients. Max limit count = 5"
40 );
41 Field<ListGraphType<IntGraphType>>(
42 "availableTransactionYears",
43 resolve: context => transactionService.GetAvailableTransactionYears(),
44 description: "Get the list of years where transactions happened"
45 );
46 Field<ListGraphType<TransactionSummaryByMonthType>>(
47 "transactionSummaryByMonth",
48 arguments: new QueryArguments(new QueryArgument<NonNullGraphType<IntGraphType>> { Name = "year" }),
49 resolve: context => transactionService.GetTransactionSummaryByMonth(context.GetArgument<int>("year")),
50 description: "Get transaction summary for a year by month"
51 );
52 }
53 }
54}
55
Mutation
1using Demo.Server.Core.BusinessEntity;
2using Demo.Server.Core.GraphQl.Types;
3using Demo.Server.Core.Service.Interface;
4using GraphQL.Types;
5using System;
6
7namespace Demo.Server.Core.GraphQl.Schema
8{
9 public class DemoMutation : ObjectGraphType<object>
10 {
11 public DemoMutation(IFeedbackService feedbackService)
12 {
13 Name = "Mutation";
14 Field<FeedbackType>(
15 "addFeedback",
16 description: "Submit feedback to the application",
17 arguments: new QueryArguments(new QueryArgument<NonNullGraphType<StringGraphType>> { Name = "text" }),
18 resolve: context =>
19 {
20 var feedbackInput = context.GetArgument<string>("text");
21 return feedbackService.AddFeedback(new Feedback(Guid.NewGuid().ToString(), feedbackInput));
22 }
23 );
24 }
25 }
26}
27
Subscription
1using Demo.Server.Core.BusinessEntity;
2using Demo.Server.Core.GraphQl.Types;
3using Demo.Server.Core.Service.Interface;
4using GraphQL.Resolvers;
5using GraphQL.Subscription;
6using GraphQL.Types;
7using System;
8
9namespace Demo.Server.Core.GraphQl.Schema
10{
11 public class DemoSubscription: ObjectGraphType<object>
12 {
13 private readonly IFeedbackEventService _feedbackEventService;
14 public DemoSubscription(IFeedbackEventService feedbackEventService)
15 {
16 _feedbackEventService = feedbackEventService;
17
18 Name = "Subscription";
19 AddField(new EventStreamFieldType
20 {
21 Name = "feedbackEvent",
22 Type = typeof(FeedbackEventType),
23 Resolver = new FuncFieldResolver<FeedbackEvent>(ResolveEvent),
24 Subscriber = new EventStreamResolver<FeedbackEvent>(Subscribe)
25 });
26 }
27
28 private FeedbackEvent ResolveEvent(ResolveFieldContext context)
29 {
30 return context.Source as FeedbackEvent;
31 }
32
33 private IObservable<FeedbackEvent> Subscribe(ResolveEventStreamContext context)
34 {
35 return _feedbackEventService.EventStream();
36 }
37 }
38}
39
It is a graph basically. So, all the types exposed in Query subsequently will also have to inherit a GraphType. If we take a look at the first field in the Query type for example, it is a ListGraphType
1Field<ListGraphType<TransactionType>>
2(
3 "transactions",
4 arguments: new QueryArguments(new QueryArgument<NonNullGraphType<IntGraphType>> { Name = "year" }),
5 resolve: context => transactionService.GetTransactions(context.GetArgument<int>("year")),
6 description: "Get all transactions for the specified year"
7);
8
As you can see, the fields are defined in the constructor of a class. In my query example, the fields are constructed via Field method. In my subscription example, the field is constructed via AddField method. There are also other methods available to construct the fields. The library probably uses Assembly info and Reflection methods to construct the Graph in runtime. Just my rough guess though. Would definitely dig into their codes in future, if I find the time to do it 🙂
That's about it for the Schema constructions!
6. Write and Test GraphQL queries with GraphiQL
GraphiQL. What a cheeky and creative name! It's pronounced as "Graphical". Fair enough, it is the IDE for GraphQL, the place where you wanna construct, execute and test your GraphQL queries.
Think of it this way. In REST, we usually test our APIs using tools like Swagger or Postman. In GraphQL, we test it using GraphiQL. Though, there are also other tools available in the market like GraphQL Voyager, GraphQL Playground, etc. But baseline is, they are all essentially GraphQL test clients.
Let's take a look at it! It's a pretty simple and straight-forward UI. From the left, we have the history list of queries that we have executed. Followed by the Query Editor and Variables section underneath, then the results pane. We can just simply start by typing something on the editor, press Ctrl + Space to get some intellisense and autofill capabilities. When you are done, just press Ctrl + Enter or click the play button on top, the query will then be executed against the GraphQL Server to retrieve results. Pretty cool eh? 😛
This works and feels pretty much the same like using some SQL test clients, where you write your queries, hit the execute button and wait for the results to be returned. I thought it was a pretty cool paradigm shift as a web developer, from having to key in endpoints and parameters, to writing queries and variables.
On the right most pane, we have the document explorer. It contains all the information that we have written in our Schema earlier on, i.e. the field names, descriptions, etc. are all display here.
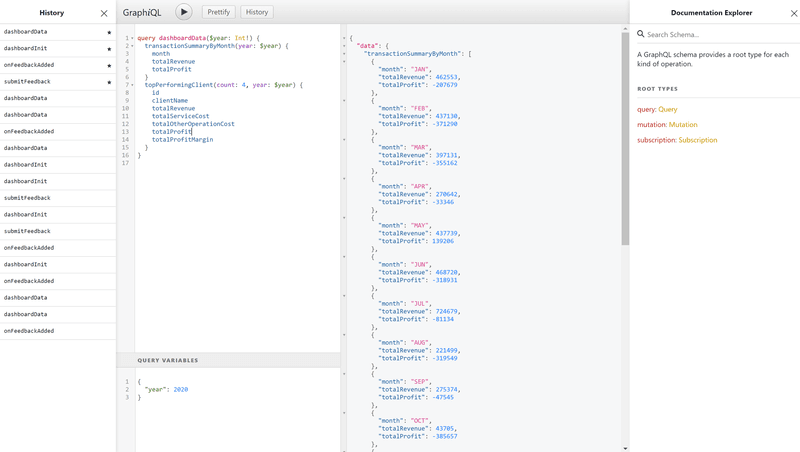
If you think about it, it's a really good collaboration tool if you are in a situation where you have a Frontend team and a Backend team. Backend team can focus on providing data, elaborate information in the "graph", where else Frontend team can then use GraphiQL to test and find the data that suits their needs. Sweet!
Not just that. After the Frontend dev is satisfied with his/her query construction. He/She can then copy and paste the query into the Frontend project directly. This is especially true if we are using a GraphQL Client package like Apollo Client, which really helps streamlining the dev process and makes the dev experience a breeze!
Great, let's get on with the next part, enter GraphQL Client!
I thought it was a pretty cool paradigm shift as a web developer, from having to key in endpoints and parameters, to writing queries and variables.
7. React + Apollo Client Integration
GraphQL Client is no more than a helper for a Client application to communicate with a GraphQL Server. It should abstract away all the "infrastructure" codes, especially things like endpoint names, enabling developers to focus just on writing GraphQL queries and fulfilling their application's needs.
In my demo example, I am using Apollo Client, which is a very popular GraphQL Client package. It is normally used along with ReactJS, but make no mistake, it is framework/library agnostic and actually works well with others framework/library like Angular, Vue, etc. too.
To setup ApolloClient, we just need an instance of it (configurations are defined here, e.g. GraphQL Server address, etc.), then wrap the portion of React tree that you want to use Apollo with ApolloProvider, with the instance as a Props.
ApolloClient Instance
1import {
2 ApolloClient,
3 InMemoryCache,
4 HttpLink,
5 split,
6} from '@apollo/client';
7import { getMainDefinition } from '@apollo/client/utilities'
8import { WebSocketLink } from '@apollo/client/link/ws';
9import { OperationDefinitionNode } from 'graphql';
10
11
12const httpLink = new HttpLink({
13 uri: "https://localhost:44391/graphql",
14});
15
16// Create a WebSocket link:
17const wsLink = new WebSocketLink({
18 uri: "wss://localhost:44391/graphql",
19 options: {
20 reconnect: true
21 }
22});
23
24const link = split(
25 // split based on operation type
26 ({ query }) => {
27 const definition = getMainDefinition(query);
28 return definition.kind === 'OperationDefinition' && (definition as OperationDefinitionNode).operation === 'subscription';
29 },
30 wsLink,
31 httpLink,
32);
33
34// Instantiate client
35const ApolloClientInstance = new ApolloClient({
36 link,
37 cache: new InMemoryCache()
38})
39
40export default ApolloClientInstance;
41
ApolloProvider
1import Admin from './Pages/Admin';
2import ApolloClientInstance from './Utils/ApolloClientInstance';
3import Dashboard from './Pages/Dashboard';
4import Feedback from './Pages/Feedback';
5import Header from './Components/Header';
6import React from 'react';
7import ReactDOM from 'react-dom';
8import { ApolloProvider } from '@apollo/client';
9import { BrowserRouter as Router, Route, Switch } from 'react-router-dom';
10import { SnackbarProvider } from 'notistack';
11import './index.scss';
12
13ReactDOM.render(
14 <ApolloProvider client={ApolloClientInstance}>
15 <SnackbarProvider maxSnack={3}>
16 <Router>
17 <Header />
18 <div className="pageContainer">
19 <Switch>
20 <Route exact path="/">
21 <Dashboard />
22 </Route>
23 <Route path="/feedback">
24 <Feedback />
25 </Route>
26 <Route path="/admin">
27 <Admin />
28 </Route>
29 </Switch>
30 </div>
31 </Router>
32 </SnackbarProvider>
33 </ApolloProvider>,
34 document.getElementById('root')
35);
36
Cool, we have now finished setting up our GraphQL Client. Let's take a look at some GraphQL queries in action!
Let's take the Dashboard page as an example here. After I am done testing with GraphiQL, I proceeded to copy the query over and assign it to GET_DASHBOARD_DATA const. The gql function is provided by the @apollo/client package.
1const GET_DASHBOARD_DATA = gql`
2 query dashboardData($year: Int!) {
3 transactionSummaryByMonth(year: $year){
4 month,
5 totalRevenue,
6 totalProfit,
7 }
8 topPerformingClient(count: 4, year: $year){
9 id
10 clientName
11 totalRevenue
12 totalServiceCost
13 totalOtherOperationCost
14 totalProfit
15 totalProfitMargin
16 }
17 }
18`;
19
The query const is then used as a parameter for the useQuery/useLazyQuery hook, depending on your usage purposes. Simple as that 😉
1const Dashboard = (): tsx.Element => {
2 const classes = useStyles();
3 const [year, setYear] = React.useState<number>(0);
4 const { data, loading, error } = useQuery<IDashboardInitQueryRes>(GET_DASHBOARD_INIT);
5 const [getData, { data: queryData }] = useLazyQuery<IDashboardDataQueryRes>(GET_DASHBOARD_DATA);
6
7 const handleChange = (event: React.ChangeEvent<{ value: unknown }>) => {
8 setYear(event.target.value as number);
9 getData({ variables: { year: event.target.value } });
10 };
11
12 const renderDataDisplay = (): tsx.Element[] => {
13 const elements = [];
14
15 if (queryData) {
16 if (queryData.transactionSummaryByMonth && queryData.transactionSummaryByMonth.length > 0) {
17 elements.push(<TransactionSummary key="transaction-summary" data={queryData.transactionSummaryByMonth} />);
18 }
19 if (queryData.topPerformingClient && queryData.topPerformingClient.length > 0) {
20 elements.push(<TopPerformingClients key="top-performing-client" data={queryData.topPerformingClient} />);
21 }
22 }
23
24 return elements;
25 };
26
27 const renderNoRecord = () => {
28 return (
29 <Typography variant="subtitle2" className={classes.noRecords}>
30 No Records
31 </Typography>
32 )
33 }
34
35 if (loading) return <p>LOADING...</p>
36 if (error) return <p>ERROR</p>;
37 if (!data) return <p>No Records</p>;
38
39 return (
40 <div className={styles['dashboard']}>
41 <FormControl className={classes.formControl}>
42 <InputLabel id="demo-simple-select-label">Year</InputLabel>
43 <Select
44 labelId="dashboard-year-select"
45 id="dashboard-year-select-id"
46 value={year}
47 onChange={handleChange}
48 >
49 {data.availableTransactionYears.map((year, index) => {
50 return <MenuItem key={index} value={year}>{year}</MenuItem>;
51 })}
52 </Select>
53 </FormControl>
54 {!queryData && renderNoRecord()}
55 {renderDataDisplay()}
56 </div>
57 );
58};
59
Okay! I have covered most of important stuffs by now
8. Demo - Query, Mutation and Subscription in Action!
Alright, demo time! I have setup 3 pages for this demo, covering different parts of GraphQL
- Dashboard -> Query
- Feedback -> Mutation
- Admin -> Subscription
9. Caveat!
If you using Windows, you will probably run into the problem of not being able to get the Subscription to work. There could be either 2 reasons to this. You are using Windows 7 (or any version before 8) or you did not turn on the WebSocket protocol on your Windows Feature.
If you are using Windows 7, then you probably won't be able to get it works since Windows 7 does not support WebSocket by default. There could be workarounds but I would not dive into it unless strictly necessary, cause it's just a real pain in the ass! 😛
If you are using Windows 8 and above, lucky you! You just need to go to Windows Feature > Internet Information Services > World Wide Web Services > Application Development Features and turn on the "WebSocket Protocol".
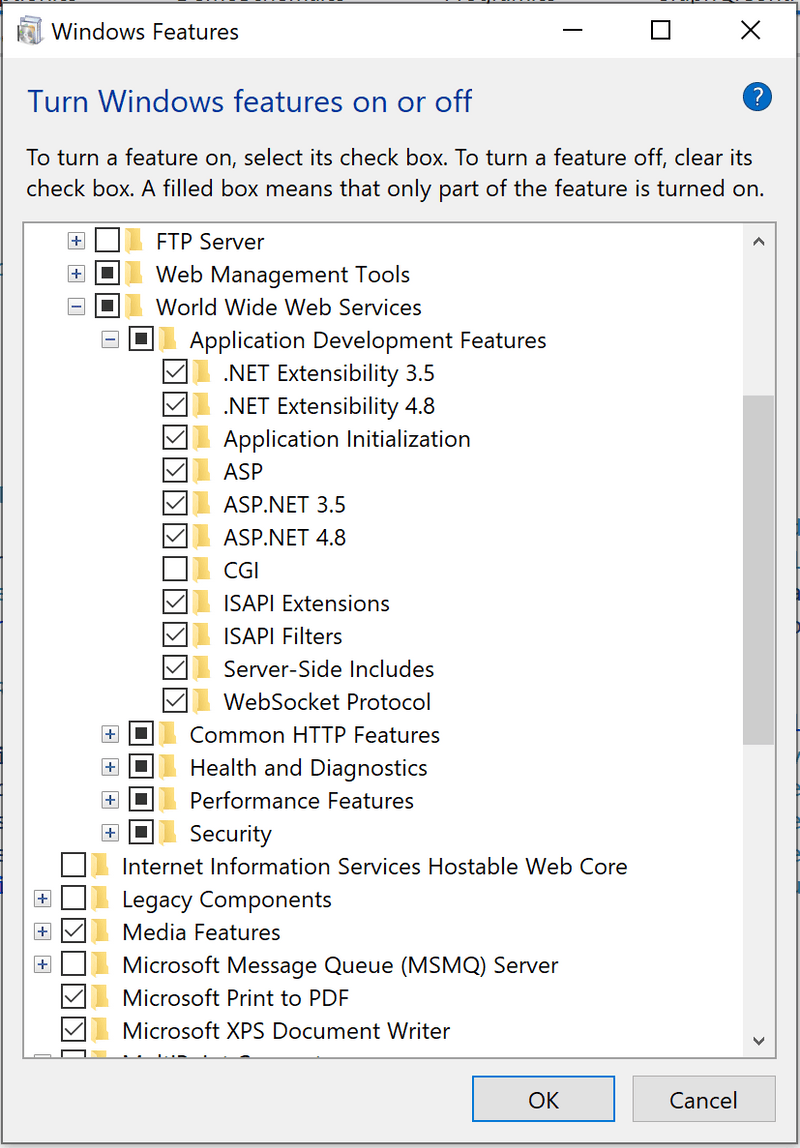
It took me some time to realize this. A silly mistake indeed. So, please don't make the same mistake like I do!
Hope that you enjoyed this one! The longest article that I have written to date. Hahaha..